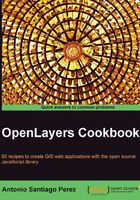
Managing map's controls
OpenLayers comes with lots of controls to interact with the map: pan, zoom, show overview map, edit features, and so on.
In the same way as layers, the OpenLayers.Map
class has methods to manage the controls attached to the map.

How to do it...
Follow the given steps:
- Create a new HTML file and add the OpenLayers dependencies.
- Now, add the required code to create the buttons and
div
element to hold the map instance:<div class="sample_menu" dojoType="dijit.MenuBar"> <span class="title">Controls: </span> <div dojoType="dijit.form.ToggleButton" iconClass="dijitCheckBoxIcon" onChange="updateMousePosition">MousePosition </div> <div dojoType="dijit.form.ToggleButton" iconClass="dijitCheckBoxIcon" onChange="updatePanPanel">PanPanel</div> <div dojoType="dijit.form.ToggleButton" iconClass="dijitCheckBoxIcon" onChange="updateZoomPanel">ZoomPanel</div> </div> <!-- Map DOM element --> <div id="ch1_managing_controls" style="width: 100%; height: 500px;"></div>
- Within the
script
element section, create the map instance:var map = new OpenLayers.Map("ch1_managing_controls", { controls: [ new OpenLayers.Control.Navigation() ] });
- Add some layers to the map and center the view:
var wms = new OpenLayers.Layer.WMS("OpenLayers WMS Basic", "http://vmap0.tiles.osgeo.org/wms/vmap0", { layers: 'basic' }, { wrapDateLine: false }); map.addLayer(wms); // Center the view map.setCenter(OpenLayers.LonLat.fromString("0,0"),3);
- Finally, add the actions code associated to the buttons:
function updateMousePosition(checked) { if(checked) { map.addControl(new OpenLayers.Control.MousePosition()); } else { var controls = map.getControlsByClass ("OpenLayers.Control.MousePosition"); console.log(controls); map.removeControl(controls[0]); } } function updatePanPanel(checked) { if(checked) { map.addControl(new OpenLayers.Control.PanPanel()); } else { var controls = map.getControlsByClass ("OpenLayers.Control.PanPanel"); map.removeControl(controls[0]); } } function updateZoomPanel(checked) { if(checked) { // Place Zoom control at specified pixel map.addControl(new OpenLayers.Control.ZoomPanel() , new OpenLayers.Pixel(50,10)); } else { var controls = map.getControlsByClass ("OpenLayers.Control.ZoomPanel"); map.removeControl(controls[0]); } }
Every button action function checks if the toggle button is checked or unchecked and depending on the value we add or remove the control to the map:
if(checked) { // Place Zoom control at specified pixel map.addControl(new OpenLayers.Control.ZoomPanel(), new OpenLayers.Pixel(50,10)); } else { var controls = map.getControlsByClass("OpenLayers.Control.ZoomPanel"); map.removeControl(controls[0]); }
Adding a control is fairly simple through the map.addControl()
method, which, given a control instance—and, optionally a OpenLayers.Pixel
instance—adds the control to the map at the specified position.
To remove a control we need to have a reference to the instance that has to be removed. The method map.getControlsByClass()
returns an array of controls of the specified class and helps us to get a reference to the desired control. Next, we can remove it with map.removeControl().
Note, in this recipe we have centered the map's view passing a OpenLayers.LonLat
instance created in a different way. Instead of using the new
operator, we have used the method OpenLayers.LonLat.fromString
, which created a new instance from a string:
map.setCenter(OpenLayers.LonLat.fromString("0,0"),3);
In addition, the map instance created in this recipe has initialized with only one control, OpenLayers.Control.Navigation()
, which allows us to navigate the map using the mouse:
var map = new OpenLayers.Map("ch1_managing_controls", { controls: [ new OpenLayers.Control.Navigation() ] });
Note
Passing an empty array to the controls
property creates a map instance without any control associated with it. In addition, without specifying the controls
property, OpenLayers creates a set of default controls for the map, which includes the OpenLayers.Control.Navigation
and OpenLayers.Control.PanZoom
controls.